Overview

When a Series is exported, the X and Y values of all data points in the series are persisted to the in-memory cache (DataSet object), where they can easily be used for the following purposes:
- Data-binding.
- Saving into a file, or stream.
- Converting to a different format, like XML.
- Editing data.
Output Data Formats
One of the main classes of the ADO.NET architecture, the DataSet class, is used to store the exported data. First, a DataTable object is created for each series, then it is added to the Tables collection of the DataSet class. Each DataTable object has the same name as the series it represents. Each DataTable object will have two, or more, columns including one column for the X value, and one, or more, columns for the Y values. Columns of the DataTable are named after the values they are storing ("X", "Y", "Y2", "Y3", etc.).
The data type of each column depends on the data point type. Data point type of a column may be a DateTime, string, or double.
Note |
The XValueType, and YValueType properties determine the data point type. If these properties are set to Auto, then the type is automatically determined by the control. |
Each data point of the series will be stored in the DataRow object, and will be inserted into the Rows collection of the DataTable.
Note |
A string value can be assigned to the X values of the points. In this case, the string is stored into the AxisLabel property, and the XAxisType property of the series is set to string. When exporting the X values of type string, the AxisLabel property must be used to access the value. |
Alternate Output Formats for Data
Using the DataSet object is the most generic and convenient way of storing structured data in memory, and you can easily convert data that is stored in a DataSet object into any format required.
Example
This example demonstrates how to convert a DataSet object into XML, and then write it to a file.
Visual Basic |
Copy Code |
Imports Dundas.Charting.WinControl
...
' Create new series.
Dim mySeries As New Series("MySeries")
' Add series points.
mySeries.Points.AddXY(2.0F, 7.0F)
mySeries.Points.AddXY(7.0F, 15.0F)
mySeries.Points.AddXY(9.0F, 4.0F)
' Exporting series using the Series object.
Dim seriesData As DataSet = Chart1.DataManipulator.ExportSeriesValues(mySeries)
' Saving exported data as an XML file.
seriesData.WriteXml("mySeriesData.xml")
|
C# |
Copy Code |
using Dundas.Charting.WinControl;
...
// Create new series.
Series mySeries = new Series("MySeries");
// Add series points.
mySeries.Points.AddXY(2.0F, 7.0F);
mySeries.Points.AddXY(7.0F, 15.0F);
mySeries.Points.AddXY(9.0F, 4.0F);
// Exporting series using the Series object.
DataSet seriesData = Chart1.DataManipulator.ExportSeriesValues(mySeries);
// Saving exported data as an XML file.
seriesData.WriteXml("mySeriesData.xml");
|
Exporting Series
The X and Y values of one or more series can be exported using the ExportSeriesValues method of the DataManipulator object. Calling this method without specifying one or more series will, by default, export all values from all series objects in the chart series collection. If you want to export the data from specific series you can specify them by name or by using a Series object.
Example
This example demonstrates how to export all, one, or several series.
Visual Basic |
Copy Code |
Imports Dundas.Charting.WinControl
...
' Exporting all series in the chart series collection.
Dim allSeriesData As DataSet = Chart1.DataManipulator.ExportSeriesValues()
' Exporting two series from the chart series collection by names.
Dim twoSeriesData As DataSet = Chart1.DataManipulator.ExportSeriesValues("MySeries1, MySeries2")
' Exporting series using the Series object.
Dim mySeries As New Series("MySeries")
mySeries.Points.AddXY(2.0F, 7.0F)
mySeries.Points.AddXY(7.0F, 15.0F)
mySeries.Points.AddXY(9.0F, 4.0F)
Dim seriesData As DataSet = Chart1.DataManipulator.ExportSeriesValues(mySeries)
|
C# |
Copy Code |
using Dundas.Charting.WinControl;
...
// Exporting all series in the chart series collection.
DataSet allSeriesData = Chart1.DataManipulator.ExportSeriesValues();
// Exporting two series from the chart series collection by names.
DataSet twoSeriesData = Chart1.DataManipulator.ExportSeriesValues("MySeries1, MySeries2");
Series mySeries = new Series("MySeries");
// Exporting series using the Series object.
mySeries.Points.AddXY(2.0F, 7.0F);
mySeries.Points.AddXY(7.0F, 15.0F);
mySeries.Points.AddXY(9.0F, 4.0F);
DataSet seriesData = Chart1.DataManipulator.ExportSeriesValues(mySeries);
|
Exporting Data
Data points in a series may be marked as Empty. This means that there is no real Y value assigned to the data point. While exporting the data the empty points can be either ignored or exported as DBNULL values, depending on the IgnoreEmptyPoints property of the DataManipulator object. If IgnoreEmptyPoints is set to true then empty points are not exported. If it is False the empty data points will be stored as DBNull objects in the DataRow object.
Example
This example demonstrates how to data-bind a DataGrid control to an exported series. We assume that a DataGrid and DataSet objects were added to the Windows Forms page. Also, we assume that a DataGrid event was added for the modification and changing of data points. Finally, we assume that the DundasBlue template has been applied to the original chart for appearance purposes. The result of the data-binding operation is shown in Figure 1 below.
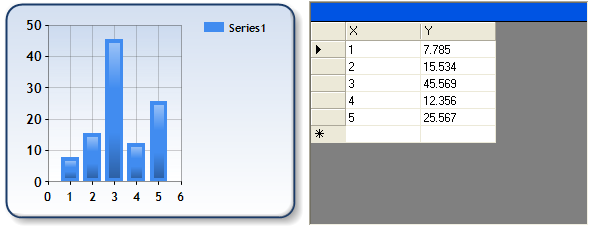
Figure 1: The databound chart.
Visual Basic |
Copy Code |
Imports Dundas.Charting.WinControl
...
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs)
' add data to series.
chart1.Series("Series1").Points.AddY(7.785)
chart1.Series("Series1").Points.AddY(15.534)
chart1.Series("Series1").Points.AddY(45.569)
chart1.Series("Series1").Points.AddY(12.356)
chart1.Series("Series1").Points.AddY(25.567)
' Export series values into DataSet object
dataset = chart1.DataManipulator.ExportSeriesValues()
' Data bind DataGrid control.
dataGrid1.DataSource = dataset
' Set Series name for data
dataGrid1.DataMember = "Series1"
End Sub
Private Sub dataGrid1_CurrentCellChanged(ByVal sender As Object, ByVal e As System.EventArgs)
' Initializes a new instance of the DataView class
Dim firstView As DataView = New DataView(dataset.Tables(0))
' Since the DataView implements IEnumerable, pass the reader directly into
' the DataBind method with the name of the Columns selected in the query
chart1.Series("Series1").Points.DataBindXY(firstView, "X", firstView, "Y")
' Invalidate Chart
chart1.Invalidate()
End Sub
|
C# |
Copy Code |
using Dundas.Charting.WinControl;
...
private void Form1_Load(object sender, EventArgs e)
{
// add data to series.
chart1.Series["Series1"].Points.AddY(7.785);
chart1.Series["Series1"].Points.AddY(15.534);
chart1.Series["Series1"].Points.AddY(45.569);
chart1.Series["Series1"].Points.AddY(12.356);
chart1.Series["Series1"].Points.AddY(25.567);
// Export series values into DataSet object
dataset = chart1.DataManipulator.ExportSeriesValues();
// Data bind DataGrid control.
dataGrid1.DataSource = dataset;
// Set Series name for data
dataGrid1.DataMember = "Series1";
}
private void dataGrid1_CurrentCellChanged(object sender, System.EventArgs e)
{
// Initializes a new instance of the DataView class
DataView firstView = new DataView(dataset.Tables[0]);
// Since the DataView implements IEnumerable, pass the reader directly into
// the DataBind method with the name of the Columns selected in the query
chart1.Series["Series1"].Points.DataBindXY(firstView, "X", firstView, "Y");
// Invalidate Chart
chart1.Invalidate();
}
|
Example
This example demonstrates how to export series data, filter the data, and then bind the data to a DataView object. We assume that "Series1" exists in the series collection. Further, we assume that the DundasBlue template has been applied to the original chart for appearance purposes.
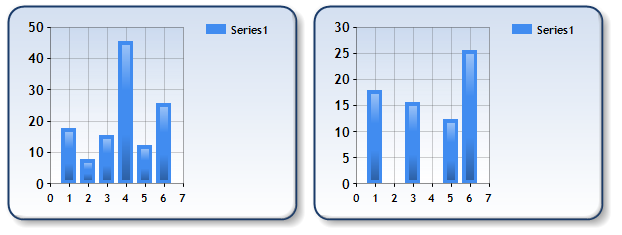
Figure 3: The series before(left) and after(right) data-binding to the filtered series data.
Visual Basic |
Copy Code |
Imports Dundas.Charting.WinControl
...
' Populate table with the series data in the data set.
chart1.Series("Series1").Points.AddXY(1, 17.785)
chart1.Series("Series1").Points.AddXY(2, 7.785)
chart1.Series("Series1").Points.AddXY(3, 15.534)
chart1.Series("Series1").Points.AddXY(4, 45.569)
chart1.Series("Series1").Points.AddXY(5, 12.356)
chart1.Series("Series1").Points.AddXY(6, 25.567)
' Exporting series using the Series object.
Dim seriesData As DataSet = chart1.DataManipulator.ExportSeriesValues("Series1")
' Get default data view of the series data.
Dim defaultView As DataView = seriesData.Tables("Series1").DefaultView
' Filter all points with Y values less then 10 and more than 25.
defaultView.RowFilter = "Y > 10 And Y < 30"
' Databind series to the filtered view.
chart1.Series("Series1").Points.DataBindXY(defaultView, "X", defaultView, "Y")
|
C# |
Copy Code |
using Dundas.Charting.WinControl
...
// Populate table with the series data in the data set.
chart1.Series["Series1"].Points.AddXY(1, 17.785);
chart1.Series["Series1"].Points.AddXY(2, 7.785);
chart1.Series["Series1"].Points.AddXY(3, 15.534);
chart1.Series["Series1"].Points.AddXY(4, 45.569);
chart1.Series["Series1"].Points.AddXY(5, 12.356);
chart1.Series["Series1"].Points.AddXY(6, 25.567);
// Exporting series using the Series object.
DataSet seriesData = chart1.DataManipulator.ExportSeriesValues("Series1");
// Get default data view of the series data.
DataView defaultView = seriesData.Tables["Series1"].DefaultView;
// Filter all points with Y values less then 10 and more than 25.
defaultView.RowFilter = "Y > 10 And Y < 30";
// Databind series to the filtered view.
chart1.Series["Series1"].Points.DataBindXY(defaultView, "X", defaultView, "Y");
|
See Also